Tabs
Allows users to create a layout with multiple tabs.
app.tabs
app.tabs(tab_key, disabled=False, icon=None, label=None, initial_tab=None, size=None,
tab_position=None, type=None)
Parameters
Parameter | Description |
---|---|
tab_key (str) | The key of the tab, used to identify each tab. |
disabled (bool) | Disables the tab. |
icon (IconType) | Specifies an icon to be displayed in the tab header. |
label (str) | Text label displayed on the tab header. |
initial_tab (Optional[str]) | The key of the tab that should be active initially. |
size (TabsSize) | Size of the tabs, which can be 'large', 'middle', or 'small'. |
tab_position (TabsPosition) | Position of the tabs to the tab content, such as 'top', 'right', 'bottom', or 'left'. |
type (TabsType) | Type of tabs. |
Examples
The basic examples below shows how to create a Tabs layout widget.
Example 1 - Basic Tabs Layout
from shapelets.apps import IconCodes, TabParams, dataApp
# Set up the data app
app = dataApp()
# Create Tabs with simple text labels
tab1, tab2, tab3 = app.tabs(
[
{'tab_key': 'tab1', 'label': 'Tab 1'},
{'tab_key': 'tab2', 'label': 'Tab 2'},
{'tab_key': 'tab3', 'label': 'Tab 3'}
]
)
tab1.text("Hello, World!")
tab2.text("Welcome to Shapelets!")
tab3.text("Enjoy working with Shapelets!")
- Below are the images of the Tabs layout widget, where you can access the content of each tab by clicking on the respective tab header.
Example 2 - Basic Tabs with Sliders + Rating
from shapelets.apps import IconCodes, TabParams, dataApp, serve
app = dataApp()
tab1, tab2 = app.tabs(2)
tab1.label = 'Tab 1'
tab1.slider()
tab2.label = 'Tab 2'
tab2.icon = IconCodes.SearchOutlined
tab2.rate()
tab1_spec: TabParams = {'tab_key': 'apple', 'icon': IconCodes.AppleFilled, 'label': 'Apple'}
tab2_spec: TabParams = {'tab_key': 'android', 'icon': IconCodes.AndroidFilled, 'label': 'Android'}
tab1, tab2 = app.tabs([tab1_spec, tab2_spec], initial_tab="android")
tab1.text("Pick Apple")
tab2.text("Pick Android")
if __name__ == '__main__':
import json
print(json.dumps(app._to_wire(), indent=4))
serve(app, deploy_ui=False, port=5001)
- Below are the images of the Tabs layout widget, where you can access the content of each tab by clicking on the respective tab header.
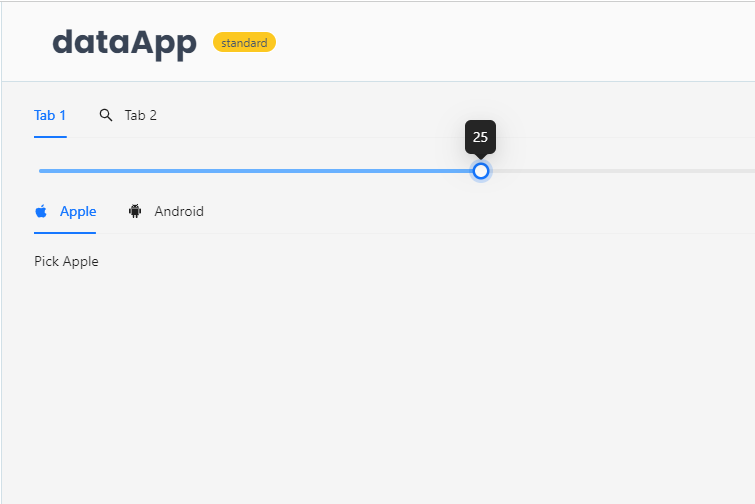
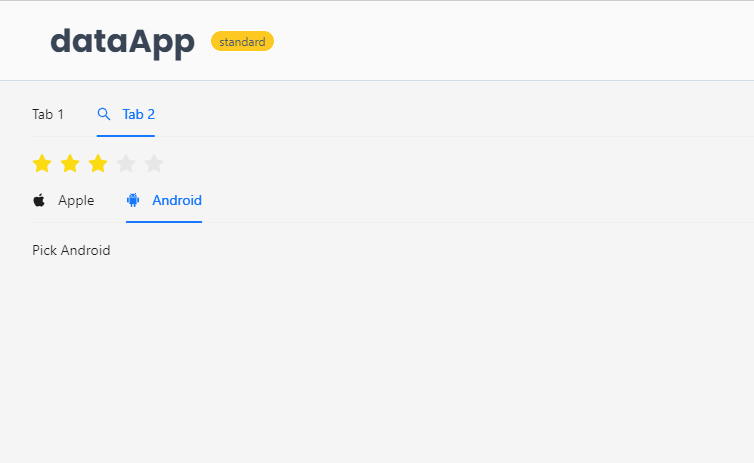